JavaScript Volume 1
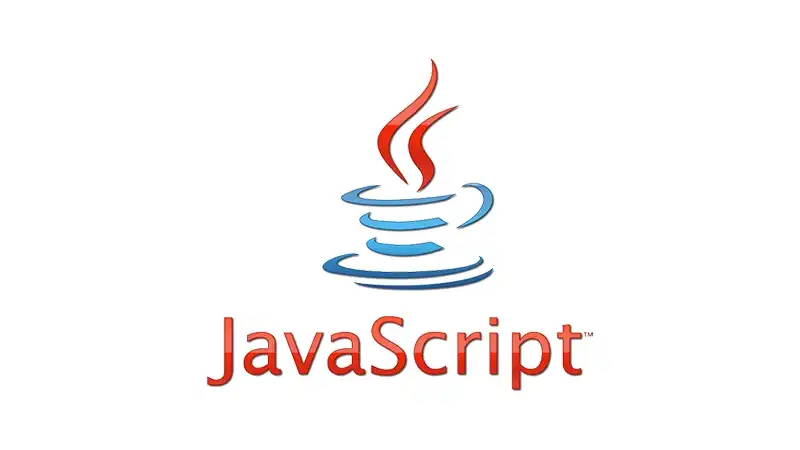
版权声明:署名-非商业性使用-相同方式共享
@@ Tags: JavaScript;语法;函数;对象;属性;原型;迭代器
@@ Date: 2023-03-07 1937
JavaScript Introduction
JavaScript is the world's most popular programming language.
JavaScript is the programming language of the Web.
JavaScript is easy to learn.
JavaScript and Java are completely different languages, both in concept and design.
JavaScript was invented by Brendan Eich in 1995, and became an ECMA standard in 1997.
ECMA-262 is the official name of the standard. ECMAScript is the official name of the language.
// 改变HTML内容
document.getElementById("demo").innerHTML = "Hello JavaScript";
// 改变属性值
document.getElementById('myImage').src='pic_bulboff.gif'
// 改变CSS样式
document.getElementById("demo").style.fontSize = "35px";
JavaScript 位置
Scripts can be placed in the <body>
, or in the <head>
section of an HTML page, or in both.
<script>
document.getElementById("demo").innerHTML = "My First JavaScript";
</script>
<script src="myScript.js"></script>
<script src="https://www.w3schools.com/js/myScript.js"></script>
JavaScript 输出
JavaScript can "display" data in different ways:
innerHTML
Writing into an HTML element, the innerHTML property defines the HTML contentdocument.write()
- Writing into the HTML output(写入到HTML文档末尾).
- Using
document.write()
after an HTML document is loaded, will delete all existing HTML.
window.alert()
Writing into an alert box.console.log()
Writing into the browser console.window.print()
- JavaScript does not have any print object or print methods.
- You cannot access output devices from JavaScript.
- The only exception is that you can call the window.print() method in the browser to print the content of the current window.
JavaScript Statements(语句)
A computer program is a list of "instructions" to be "executed" by a computer.
In a programming language, these programming instructions are called statements.
A JavaScript program is a list of programming statements.
let x, y, z; // Statement 1
x = 5; // Statement 2
y = 6; // Statement 3
z = x + y; // Statement 4
In HTML, JavaScript programs are executed by the web browser.
JavaScript statements are composed(构成) of:
Values, Operators, Expressions, Keywords, and Comments.
Semicolons ";"
Semicolons separate JavaScript statements.
When separated by semicolons, multiple statements on one line are allowed:
a = 5; b = 6; c = a + b;
On the web, you might see examples without semicolons.
Ending statements with semicolon is not required, but highly recommended.JavaScript ignores multiple spaces. You can add white space to your script to make it more readable.
If a JavaScript statement does not fit on one line, the best place to break it is after an operator.
JavaScript Keywords
@@ Refer: https://www.w3schools.com/js/js_reserved.asp
JavaScript keywords are reserved words. Reserved words cannot be used as names for variables.
- var
- let
- const
- if
- switch
- for
- function
- return
- try
JavaScript Syntax(语法)
JavaScript Values
The JavaScript syntax defines two types of values:
- Literals(字面量)
- Fixed values
- Numbers
10.50
1001
- Strings
"John Doe"
'John Doe'
- Variables(可变值)
- Variable values, variables are used to store data values.
- JavaScript uses the keywords
var
,let
andconst
to declare variables. - An equal sign is used to
assign values
(赋值) to variables. - Example:
let x; x = 6;
JavaScript Expressions(表达式)
An expression is a combination(组合) of values, variables, and operators, which computes to a value.
The computation is called an evaluation(求值).
5 * 10
JavaScript Comments(注释)
Not all JavaScript statements are "executed".
Code after double slashes //
or between /*
and */
is treated as a comment.
let x = 5; // I will be executed
// x = 6; I will NOT be executed
JavaScript Identifiers / Names(标识符命名)
Identifiers are used to name variables and keywords, and functions.
A JavaScript name must begin with:
- A letter (A-Z or a-z)
- A dollar sign ($)
- Or an underscore (_)
Note: Numbers are not allowed as the first character in names.
JavaScript is Case Sensitive(区分大小写)
let lastname, lastName;
lastName = "Doe";
lastname = "Peterson";
JavaScript and Camel Case(驼峰命名法)
Historically, programmers have used different ways of joining multiple words into one variable name:
- Hyphens(连字符)
- first-name, last-name, master-card, inter-city.
- Underscore(下划线)
- first_name, last_name, master_card, inter_city.
- Upper Camel Case(Pascal Case, 大驼峰命名法)
- FirstName, LastName, MasterCard, InterCity.
- Lower Camel Case(小驼峰命名法)
- firstName, lastName, masterCard, interCity.
- JavaScript programmers tend to use camel case that starts with a lowercase letter
JavaScript Character Set(字符集)
JavaScript uses the Unicode character set.
Unicode covers (almost) all the characters, punctuations(标点符号), and symbols in the world.
JavaScript Variables
Variables are containers for storing data (storing data values).
4 Ways to Declare a JavaScript Variable:
- Using
var
var x = 5;
- Using nothing
- 松散模式下, 它会创建成为全局对象的属性.
- 严格模式下, 会出错.
x = 5;
- Using
let
- Variables defined with
let
cannot be Redeclared. - Variables defined with
let
must be Declared before use. - Variables defined with
let
have Block Scope. let x = 5;
- Variables defined with
- Using
const
- Variables defined with
const
cannot be Redeclared. - Variables defined with
const
cannot be Reassigned(重新赋值). - Variables defined with
const
have Block Scope. - Variables must be assigned a value when they are declared.
- Constant Objects and Arrays
const x = 5;
- Variables defined with
The let
and const
keywords were added to JavaScript in 2015.
The var
keyword is used in all JavaScript code from 1995 to 2015.
Declaring(声明) a JavaScript Variable
Creating a variable in JavaScript is called "declaring" a variable.
You declare a JavaScript variable with the var
or the let
keyword:
var carName;
// or
let carName;
After the declaration, the variable has no value (technically it is
undefined
).
let person = "John Doe", carName = "Volvo", price = 200;
// or
let person = "John Doe",
carName = "Volvo",
price = 200;
Re-Declaring(重新声明) JavaScript Variables
If you re-declare a JavaScript variable declared with var
, it will not lose its value.
var carName = "Volvo";
var carName; // 仍然为 "Volvo"
You cannot re-declare a variable declared with let or const.
This will not work:
let carName = "Volvo";
let carName; // SyntaxError: 'carName' has already been declared
JavaScript Dollar Sign $
Since JavaScript treats a dollar sign as a letter, identifiers containing $
are valid variable names:
let $ = "Hello World";
let $$$ = 2;
let $myMoney = 5;
Using the dollar sign is not very common in JavaScript, but professional programmers often use it as an alias(别名) for the main function in a JavaScript library.
In the JavaScript library jQuery, for instance(例如), the main function $
is used to select HTML elements. In jQuery $("p");
means "select all p elements".
Redeclaring Variables(重新声明变量)
var x = 10;
// Here x is 10
{
var x = 2;
// Here x is 2
}
// Here x is 2
// -------------
let x = 10;
// Here x is 10
{
let x = 2;
// Here x is 2
}
// Here x is 10
// -------------
const x = 10;
// Here x is 10
{
const x = 2;
// Here x is 2
}
// Here x is 10
JavaScript Scope(作用域)
参见: JavaScript Volume 2.md#JavaScript Scope
Let Hoisting(提升)
Variables defined with var
are hoisted to the top(提升到顶部) and can be initialized(初始化) at any time.
Meaning: You can use the variable before it is declared(意味着变量可以先使用后声明).
Variables defined with let
are also hoisted to the top of the block, but not initialized.
Meaning: Using a let
variable before it is declared will result in a ReferenceError
carName = "Volvo";
var carName;
carName = "Saab";
let carName = "Volvo";
Constant Objects and Arrays
It does not define a constant value. It defines a constant reference to a value(没有定义常量, 而是定了一个指向该值得常引用).
Because of this you can NOT:
- Reassign a constant value
- Reassign a constant array
- Reassign a constant object
But you CAN:
- Change the elements of constant array
- Change the properties of constant object
// You can create a constant array:
const cars = ["Saab", "Volvo", "BMW"];
// You can change an element:
cars[0] = "Toyota";
// You can add an element:
cars.push("Audi");
cars = ["Toyota", "Volvo", "Audi"]; // ERROR
// -----------
// You can create a const object:
const car = {type:"Fiat", model:"500", color:"white"};
// You can change a property:
car.color = "red";
// You can add a property:
car.owner = "Johnson";
car = {type:"Volvo", model:"EX60", color:"red"}; // ERROR
JavaScript Operators(操作符)
+
Addition-
Subtraction*
Multiplication/
Division%
Modulus(Division Remainder, 模, 取余)**
Exponentiation(乘方) (ES2016)++
Increment--
Decrement==
equal to!=
not equal===
equal value and equal type!==
not equal value or not equal type?
ternary operator>
greater than<
less than>=
greater than or equal to<=
less than or equal to&&
logical and||
logical or!
logical not
NOTE: Comparing two JavaScript objects with ==
and ===
always returns false.
Type Operators
typeof
Returns the type of a variableinstanceof
Returns true if an object is an instance of an object type
Bitwise Operators(按位操作)
Bit operators work on 32 bits numbers.
Any numeric operand in the operation is converted into a 32 bit number. The result is converted back to a JavaScript number.
Operator | Description | Example | Same as | Result | Decimal |
---|---|---|---|---|---|
& | AND | 5 & 1 | 0101 & 0001 | 0001 | 1 |
| | OR | 5 | 1 | 0101 | 0001 |
~ | NOT | ~ 5 | ~0101 | 1010 | 10 |
^ | XOR | 5 ^ 1 | 0101 ^ 0001 | 0100 | 4 |
<< | left shift | 5 << 1 | 0101 << 1 | 1010 | 10 |
>> | right shift | 5 >> 1 | 0101 >> 1 | 0010 | 2 |
>>> | unsigned right shift | 5 >>> 1 | 0101 >>> 1 | 0010 | 2 |
Operator Precedence(操作符优先级)
@@ Tags: 操作符优先级
@@ Refer: https://www.w3schools.com/js/js_precedence.asp
操作符优先级描述了哪些操作先执行? 哪些后执行?
列如: 100 + 50 * 3
表达式 操作符执行顺序为: 先乘除后加减
参见: https://www.w3schools.com/js/js_precedence.asp
JavaScript Data Types
JavaScript has 8 Datatypes
- String
- Number
- Bigint
- Boolean
true
,false
- Undefined
- Null
- Symbol
- Object
前7种为(Primitive)基本类型, 是不可变类型(Immutable). 而对象是可变(Mutable)类型.
The Object Datatype
- An object
- An array
- A date
JavaScript has dynamic types. This means that the same variable can be used to hold different data types.
let x; // Now x is undefined
x = 5; // Now x is a Number
x = "John"; // Now x is a String
Most programming languages have many number types, javascript are always one type: double (64-bit floating point).
JavaScript BigInt is a new datatype (2020) that can be used to store integer values that are too big to be represented by a normal JavaScript Number.
let x = BigInt("123456789012345678901234567890");
JavaScript objects are written with curly braces {}
.
const person = {firstName:"John", lastName:"Doe", age:50, eyeColor:"blue"};
JavaScript 流程控制
// The else if Statement
if (condition1) {
// block of code to be executed if condition1 is true
} else if (condition2) {
// block of code to be executed if the condition1 is false and condition2 is true
} else {
// block of code to be executed if the condition1 is false and condition2 is false
}
// The Switch Statement
// Switch cases use strict comparison (===).
// The values must be of the same type to match.
switch(expression) {
case x:
// code block
break;
case y:
// code block
break;
default:
// code block
}
// The For Loop(语法上与C语言相似)
for (let i = 0; i < cars.length; i++) {
text += cars[i] + "<br>";
}
// The For In Loop
// Each iteration returns a key (x)
const person = {fname:"John", lname:"Doe", age:25};
for (let x in person) {
person[x];
}
// The For Of Loop
// Toops through the values of an iterable object
// iterable - An object that has iterable properties.
const cars = ["BMW", "Volvo", "Mini"];
for (let x of cars) {
text += x;
}
// The While Loop
while (i < 10) {
text += "The number is " + i;
i++;
}
// The Do While Loop
do {
text += "The number is " + i;
i++;
}
while (i < 10);
// break and continue
for (let i = 0; i < 10; i++) {
if (i === 3) { continue; }
text += "The number is " + i + "<br>";
}
for (let i = 0; i < 10; i++) {
if (i === 3) { break; }
text += "The number is " + i + "<br>";
}
JavaScript Functions
A JavaScript function is a block of code designed to perform a particular(特定的) task.
A JavaScript function is executed when "something" invokes(调用) it (calls it).
// Function to compute the product of p1 and p2
function myFunction(p1, p2) {
return p1 * p2;
}
A JavaScript function is defined with the function
keyword, followed by a name, followed by parentheses ().
The parentheses(圆括号) may include parameter(参数) names separated by commas(逗号):
(parameter1, parameter2, ...)
- Function parameters are listed inside the parentheses () in the function definition(C语言中称为形参).
- Function arguments are the values received by the function when it is invoked(C语言中称为实参).
- Inside the function, the arguments (the parameters) behave as local variables.
When JavaScript reaches a return
statement, the function will stop executing.
NOTE:
A function defined as the property of an object, is called a method to the object.
A function designed to create new objects, is called an object constructor.
// A function expression can be stored in a variable
// The function is actually an anonymous function (a function without a name).
const x = function (a, b) {return a * b};
let z = x(4, 3);
// Functions can also be defined with a built-in JavaScript function constructor
// called Function().
// The example above is the same as writing:
const myFunction = new Function("a", "b", "return a * b");
let x = myFunction(4, 3);
// Functions are Objects
//
// The typeof operator in JavaScript returns "function" for functions.
// But, JavaScript functions can best be described as objects.
typeof myFunction; // Returns: function
// The arguments.length property returns the number of arguments received
// when the function was invoked:
function myFunction(a, b) {
return arguments.length;
}
// The toString() method returns the function as a string
function myFunction(a, b) {
return a * b;
}
let text = myFunction.toString(); // Returns: function myFunction(a, b) { return a * b; }
JavaScript Function Parameters
- JavaScript function definitions do not specify data types for parameters.
- JavaScript functions do not perform type checking on the passed arguments.
- JavaScript functions do not check the number of arguments received.
NOTE:
- 基本类型通过值传递: 修改对外部函数不可见
- 而对象通过引用传递: 修改对外部函数可见
// ES6 allows function parameters to have default values.
function myFunction(x, y = 10) {
return x + y;
}
myFunction(5);
// 可变参数将作为数组
function sum(...args) {
let sum = 0;
for (let arg of args) sum += arg;
return sum;
}
let x = sum(4, 9, 16, 25, 29, 100, 66, 77);
// The Arguments Object
x = sumAll(1, 123, 500, 115, 44, 88);
function sumAll() {
let sum = 0;
for (let i = 0; i < arguments.length; i++) {
sum += arguments[i];
}
return sum;
}
Call, Apply, Bind
@@ Tags: apply()
@@ Refer: https://www.w3schools.com/js/js_function_apply.asp
call()
参考Objects.what-is-thisbind()
参考Objects.what-is-thisapply()
与call()
类似, 但接收参数不同: 前者是数组, 后者单独接收.
const person = {
name: function() {
return this.firstName + " " + this.lastName;
}
fullName: function(city, country) {
return this.firstName + " " + this.lastName + "," + city + "," + country;
}
}
const person1 = {
firstName:"John",
lastName: "Doe"
}
person.name.call(person1); // This will return "John Doe":
person.name.apply(person1); // This will return "John Doe":
person.fullName.call(person1, "Oslo", "Norway");
person.fullName.apply(person1, ["Oslo", "Norway"]);
// -----------
const person = {
firstName:"John",
lastName: "Doe",
display: function () {
let x = document.getElementById("demo");
x.innerHTML = this.firstName + " " + this.lastName;
}
}
setTimeout(person.display.bind(person), 3000);
JavaScript Arrow Function
@@ Tags: 匿名函数;箭头函数;短函数
@@ Refer: https://www.w3schools.com/js/js_arrow_function.asp
Arrow functions were introduced in ES6.
hello = () => {
return "Hello World!";
}
// 等同于
hello = function() {
return "Hello World!";
}
// 如果函数只有一个语句并且返回了一个值, 则可以省略大括号
// This works only if the function has only one statement.
hello = () => "Hello World!";
// If you have parameters, you pass them inside the parentheses:
hello = (val) => "Hello " + val;
// In fact, if you have only one parameter, you can skip the parentheses as well
hello = val => "Hello " + val;
关于this
与常规函数不同的是, 箭头函数的this
总是引用到定义箭头函数的对象.
JavaScript Closures
@@ Tags: 闭包;Closure
@@ Refer: https://www.w3schools.com/js/js_function_closures.asp
All functions have access to the global scope.
In fact, in JavaScript, all functions have access to the scope "above" them(所有函数都可以访问它上面
的作用域).
const add = (function () {
let counter = 0;
return function () { // 捕获了外部函数的局部变量, 使得自己成为了一个闭包函数
counter += 1; return counter}
})();
add();
add();
add();
// the counter is now 3
NOTE:
A closure is a function having access to the parent scope, even after the parent function has closed.
JavaScript Objects
@@ Tags: js对象;属性
const person = {firstName:"John", lastName:"Doe", age:50, eyeColor:"blue"};
// Accessing object properties
person.lastName;
person["lastName"];
// object methods
const person1 = {
firstName: "John",
lastName : "Doe",
id : 5566,
age : 33,
fullName : function() {
return this.firstName + " " + this.lastName;
},
// ECMAScript 5 (ES5 2009) introduced Getter and Setters.
// Getter Accessors
get name() {
return this.firstName + " " + this.lastName;
},
// Setter Accessors
set name(value) {
[this.firstName, this.lastName] = value.split(" ");
}
};
person1.fullName(); // John Doe
person1.name; // John Doe
person1.name = "Jack Cheng";
person1.fullName(); // Jack Cheng
person1.name; // Jack Cheng
It is a common practice to declare objects with the const
keyword.
Avoid String
, Number
, and Boolean
objects. They complicate your code and slow down execution speed.
x = new String(); // Declares x as a String object
y = new Number(); // Declares y as a Number object
z = new Boolean(); // Declares z as a Boolean object
JavaScript Iterables and for..of
@@ Tags: 迭代器;可迭代对象;for of
@@ Refer: https://www.w3schools.com/js/js_object_iterables.asp
The iterator protocol defines how to produce a sequence of values from an object.
An object becomes an iterator(迭代器) when it implements a next()
method.
The next()
method must return an object with two properties:
- value
The value returned by the iterator(Can be omitted if done is true) - done
true
if the iterator has completedfalse
if the iterator has produced a new value
// Home Made Iterable
// Note: It does not support the JavaScript for..of statement.
function myNumbers() {
let n = 0;
return {
next: function() {
n += 10;
return {value:n, done:false};
}
};
}
// Create Iterable
const n = myNumbers();
n.next().value; // 10
n.next().value; // 20
n.next().value; // 30
A JavaScript iterable is an object that has a Symbol.iterator.
The Symbol.iterator
is a function that returns a next()
function.
An iterable can be iterated over with the code: for (const x of iterable) { }
// Create an Object
myNumbers = {};
// Make it Iterable
myNumbers[Symbol.iterator] = function() {
let n = 0;
done = false;
return {
next() {
n += 10;
if (n == 100) {done = true}
return {value:n, done:done};
}
};
}
// Now you can use for..of
// The Symbol.iterator method is called automatically by for..of
let text = ""
for (const num of myNumbers) {
text += num +", "
} // text: 10, 20, 30, 40, 50, 60, 70, 80, 90,
Creating a JavaScript Object
@@ Tags: js创建对象;动态生成
@@ Refer: https://www.w3schools.com/js/js_object_definition.asp
There are different ways to create new objects:
-
Create a single object, using an object literal.
const person = {firstName:"John", lastName:"Doe", age:50, eyeColor:"blue"};
-
Create a single object, with the keyword
new
.const person = new Object(); person.firstName = "John"; person.lastName = "Doe"; person.age = 50; person.eyeColor = "blue";
-
Define an object constructor, and then create objects of the constructed type.
function Person(first, last, age, eye) { // object constructor function this.firstName = first; this.lastName = last; this.age = age; this.eyeColor = eye; } const myFather = new Person("John", "Doe", 50, "blue");
-
Create an object using
Object.create()
.// 以指定对象作为原型创建新对象 // Object.create(proto [, propertiesObject]) const person1 = {firstName:"John", lastName:"Doe", age:50, eyeColor:"blue"}; const person2 = Object.create(person1);
Object Properties
@@ Tags: js对象属性;属性的属性
@@ Refer: https://www.w3schools.com/js/js_object_properties.asp
Properties are the values associated with a JavaScript object.
Property Attributes(属性的属性)
All properties have a name. In addition they also have a value.
The value is one of the property's attributes.
Other attributes are: enumerable(可枚举), configurable(可配置), and writable(可写).
Prototype(原型) Properties
All JavaScript objects inherit properties and methods from a prototype.
Date
objects inherit fromDate.prototype
Array
objects inherit fromArray.prototype
Person
objects inherit fromPerson.prototype
function Person(first, last, age, eyecolor) {
this.firstName = first;
this.lastName = last;
this.age = age;
this.eyeColor = eyecolor;
}
const myFather = new Person("John", "Doe", 50, "blue");
*
// 通过原型添加属性与方法, 影响所有的对象(包括已经创建的)
Person.prototype.nationality = "English";
Person.prototype.name = function() {
return this.firstName + " " + this.lastName;
};
myFather.nationality; // English
NOTE:
- The
Object.prototype
is on the top of the prototype inheritance chain.- The
delete
keyword does not delete inherited properties, but if you delete a prototype property, it will affect all objects inherited from the prototype.
What is this?
In JavaScript, the this
keyword refers to an object(this
是一个对象的引用).
Which object depends on how this
is being invoked (used or called).
The this
keyword refers to different objects depending on how it is used:
- In an object method,
this
refers to the object.
对于对象方法,this
是该对象的引用 - In a function,
this
refers to the global object.
对于标准函数,this
是全局object的引用, 而在严格模式(strict mode)下则是undefined
- In an event,
this
refers to the element that received the event.
对于事件,this
是接收该事件的元素的引用 - Methods like
call()
,apply()
, andbind()
can referthis
to any object.
call()
,apply()
, andbind()
可以引用this
到任何对象
Explicit Function Binding(绑定)
The call()
and apply()
methods are predefined JavaScript methods.
They can both be used to call an object method with another object as argument.
const person1 = {
firstName:"Hege",
lastName: "Nilsen",
fullName: function() {
return this.firstName + " " + this.lastName;
}
}
const person2 = {
firstName:"John",
lastName: "Doe",
}
const member = {
firstName:"Hege",
lastName: "Nilsen",
}
// 直接调用
// Return "John Doe":
person1.fullName.call(person2);
// With the bind() method, an object can borrow a method from another object.
// 返回绑定后的方法
// Return "Hege Nilsen":
person1.fullName.bind(member)();
This Precedence(优先级)
@@ Tags: this优先级
@@ Refer: https://www.w3schools.com/js/js_this.asp
To determine which object this
refers to; use the following precedence of order.
- bind()
- apply() and call()
- Object method
- Global scope
JavaScript ES5 Object Methods
// Create object with an existing object as prototype
Object.create()
// Adding or changing an object property
Object.defineProperty(object, property, descriptor)
// Adding or changing object properties
Object.defineProperties(object, descriptors)
// Accessing Properties
Object.getOwnPropertyDescriptor(object, property)
// Returns all properties as an array
Object.getOwnPropertyNames(object)
// Accessing the prototype
Object.getPrototypeOf(object)
// Returns enumerable properties as an array
Object.keys(object)
// Protecting Objects
// Prevents adding properties to an object
Object.preventExtensions(object)
// Returns true if properties can be added to an object
Object.isExtensible(object)
// Prevents changes of object properties (not values)
Object.seal(object)
// Returns true if object is sealed
Object.isSealed(object)
// Prevents any changes to an object
Object.freeze(object)
// Returns true if object is frozen
Object.isFrozen(object)
Example
// Change a property
Object.defineProperty(person, "language", {value : "NO"});
// Makes language read-only:
Object.defineProperty(person, "language", {writable:false});
// Makes language not enumerable:
Object.defineProperty(person, "language", {enumerable:false});
// Define a getter
Object.defineProperty(person, "fullName", {
get: function () {return this.firstName + " " + this.lastName;}
});
HTML Events
HTML events are "things" that happen to HTML elements(HTML事件是发生在HTML元素上的"事情").
JavaScript lets you execute code when events are detected.
HTML allows event handler attributes, with JavaScript code, to be added to HTML elements.
<button onclick="document.getElementById('demo').innerHTML = Date()">The time is?</button>
- onchange
An HTML element has been changed - onclick
The user clicks an HTML element - onmouseover
The user moves the mouse over an HTML element - onmouseout
The user moves the mouse away from an HTML element - onkeydown
The user pushes a keyboard key - onload
The browser has finished loading the page
Comments ()